Mastering Arduino Programming: A Comprehensive Beginner's Guide for Building Exciting Projects
Arduino, a popular open-source electronics platform, has gained immense popularity among hobbyists, students, and professionals alike. Its user-friendly nature and versatility make it an excellent choice for learning and implementing various projects. However, if you're new to Arduino programming, understanding the basics is crucial. In this primer, we'll explore the fundamental concepts of Arduino coding, enabling you to kickstart your journey into the exciting world of Arduino programming.
If you're new to Arduino programming, fear not! We've got your back. In this captivating primer, we'll unravel the secrets of Arduino coding, like uncovering buried treasure. Together, we'll dive deep into the fundamental concepts that will ignite a spark within you and propel you into the captivating universe of Arduino programming.
Get ready to unleash your imagination, as Arduino opens the door to a world where electronics come to life. So grab your thinking caps, fasten your seatbelts, and prepare to take off on a mind-blowing journey that will leave you inspired, awestruck, and ready to conquer the exciting world of Arduino programming!
Anatomy of an Arduino Sketch
In Arduino programming, a sketch refers to the code you write for your Arduino board. Every sketch consists of two essential functions: setup() and loop(). The setup() function is executed only once when the Arduino board powers up or is reset. It is used to initialize variables, set pin modes, and perform other one-time setup tasks. The loop() function, as the name suggests, runs continuously after the setup() function completes, allowing you to implement repetitive tasks.
Here's a simple Arduino code example that demonstrates the basic structure of an Arduino sketch with the setup() and loop() functions:
// Pin connected to an LED
const int ledPin = 13;
void setup() {
// Initialize the LED pin as an output
pinMode(ledPin, OUTPUT);
}
void loop() {
// Turn the LED on
digitalWrite(ledPin, HIGH);
// Wait for 1 second
delay(1000);
// Turn the LED off
digitalWrite(ledPin, LOW);
// Wait for 1 second
delay(1000);
}
In this code, we're using the built-in LED pin on most Arduino boards, which is pin number 13.
In the setup() function, we initialize the ledPin as an output using the pinMode() function. This tells the Arduino that we want to use the pin to control an output device, in this case, an LED.
The loop() function is where the main code execution takes place. In this example, we repeatedly turn the LED on and off at one-second intervals.
Inside the loop() function, we first use digitalWrite() to set the ledPin to HIGH, which turns the LED on. Then, we use the delay() function to pause the code execution for 1 second (1000 milliseconds).
Next, we use digitalWrite() again to set the ledPin to LOW, turning the LED off. Another delay() function is used to pause the code execution for another 1 second.
This sequence of turning the LED on and off is repeated indefinitely because the loop() function runs continuously.
When you upload this code to your Arduino board, you'll observe the LED blinking on and off every second, creating a simple flashing effect.
This basic example demonstrates how the setup() function is used for one-time initialization tasks, and the loop() function runs repeatedly, allowing you to implement repetitive actions or behaviors in your Arduino projects.
Variables and Data Types
Variables are crucial in Arduino programming. They store and manipulate data throughout your code. Arduino supports various data types, including integers, floating-point numbers, characters, and Boolean values. Understanding the appropriate use of each data type is essential for efficient programming.
In Arduino programming, variables are used to store and manipulate data. Arduino supports several data types that define the range and type of values that can be stored in a variable. Let's explore some common data types in Arduino and provide examples for each:
Integer (int):
Integers represent whole numbers without decimal points. They can be positive or negative. The int data type in Arduino is typically represented by a 16-bit signed integer, which has a range of -32,768 to 32,767.
Example:
int age = 25;
Unsigned Integer (unsigned int):
Unsigned integers represent positive whole numbers. They don't allow negative values. The unsigned int data type in Arduino is also a 16-bit integer, but it has a range of 0 to 65,535.
Example:
unsigned int count = 100;
Long (long):
Long data type is used to represent larger whole numbers. It is a 32-bit signed integer with a range of approximately -2 billion to 2 billion.
Example:
long population = 7831000000;
Unsigned Long (unsigned long):
Similar to long, the unsigned long data type represents larger positive whole numbers. It is also a 32-bit integer, but it has a range of 0 to approximately 4 billion.
Example:
unsigned long distance = 2357894321;
Floating-Point (float):
Floating-point data types are used to represent numbers with decimal points. Float variables have a precision of around 6-7 decimal digits.
Example:
float temperature = 25.5;
Double (double):
Double data type is similar to float but provides higher precision, typically around 15 decimal digits.
Example:
double pi = 3.14159265359;
Character (char):
Character data type is used to store a single character or ASCII value. Characters are enclosed in single quotes (' ').
Example:
char grade = 'A';
Boolean (bool):
Boolean data type represents a logical value, either true or false. It is commonly used for conditions and comparisons.
Example:
bool isOn = true;
These are the most commonly used data types in Arduino programming. It's important to choose the appropriate data type based on the range and type of data you need to store.
Functions
Functions are blocks of reusable code that perform specific tasks. By creating and using functions, you can break down complex problems into manageable pieces. Arduino provides built-in functions like digitalRead(), analogWrite(), and delay(). You can also define your own functions to encapsulate specific functionalities and improve code readability.
Let's say you want to blink an LED connected to pin 7 for a specific number of times. Here's an example using a custom function:
const int ledPin = 7;
void setup() {
pinMode(ledPin, OUTPUT);
}
void loop() {
blinkLED(3, 500);
}
void blinkLED(int numTimes, int interval) {
for (int i = 0; i < numTimes; i++) {
digitalWrite(ledPin, HIGH);
delay(interval);
digitalWrite(ledPin, LOW);
delay(interval);
}
}
In this code, we define a constant ledPin representing the pin number of the LED.
In the setup() function, we set ledPin as an output.
In the loop() function, we call the blinkLED() function, passing the number of times we want the LED to blink (3 times) and the interval between blinks (500 milliseconds).
The blinkLED() function takes two parameters: numTimes and interval. Inside the function, we use a for loop to iterate numTimes times. In each iteration, we turn the LED on, delay for interval milliseconds, turn the LED off, and delay again for interval milliseconds.
By calling the blinkLED() function in the loop(), the LED will blink three times with a 500-millisecond interval between each blink.
Digital Input and Output
Arduino boards have pins that can be used for digital input and output operations. By using functions like digitalRead() and digitalWrite(), you can read the state of a digital input pin or set the state of a digital output pin, respectively. This allows you to interface with switches, buttons, LEDs, and other digital devices.
Let's say you have a push-button connected to pin 2 of your Arduino board. The button is normally open, and when it's pressed, you want an LED connected to pin 13 to turn on. Here's the code:
const int buttonPin = 2;
const int ledPin = 13;
void setup() {
pinMode(buttonPin, INPUT);
pinMode(ledPin, OUTPUT);
}
void loop() {
if (digitalRead(buttonPin) == HIGH) {
digitalWrite(ledPin, HIGH);
} else {
digitalWrite(ledPin, LOW);
}
}
In this code, we define two constants: buttonPin and ledPin, representing the pin numbers for the button and LED, respectively.
In the setup() function, we set buttonPin as an input using pinMode() and ledPin as an output.
In the loop() function, we continuously check the state of the button using digitalRead(buttonPin). If the button is pressed (i.e., its state is HIGH), we turn on the LED by setting ledPin to HIGH using digitalWrite(). Otherwise, if the button is not pressed, we turn off the LED by setting ledPin to LOW.
Analog Input and Output
In addition to digital operations, Arduino also supports analog input and output. Analog inputs are useful for reading data from sensors that provide continuous variable values. Analog outputs enable you to control devices like servos and generate varying voltages using Pulse Width Modulation (PWM).
Let's say you have a potentiometer connected to analog pin A0, and you want to control the brightness of an LED connected to pin 9 based on the potentiometer's position. Here's the code:
const int potPin = A0;
const int ledPin = 9;
void setup() {
pinMode(ledPin, OUTPUT);
}
void loop() {
int potValue = analogRead(potPin);
int brightness = map(potValue, 0, 1023, 0, 255);
analogWrite(ledPin, brightness);
}
In this example, we define two constants: potPin and ledPin, representing the analog input pin and the PWM output pin for the LED, respectively.
In the setup() function, we set ledPin as an output.
In the loop() function, we read the value from the potentiometer using analogRead(potPin), which returns a value between 0 and 1023 representing the analog voltage. We then use the map() function to map the potentiometer's value to a brightness range from 0 to 255.
Finally, we use analogWrite(ledPin, brightness) to set the brightness of the LED connected to ledPin based on the potentiometer's position.
Control Structures
Control structures, such as conditional statements (if, else if, else) and loops (for, while), allow you to make decisions and repeat code blocks based on certain conditions. Understanding control structures is crucial for implementing conditional logic and iterative tasks in your Arduino projects.
If-else Statement
The if-else statement allows you to perform different actions based on a condition. If the condition is true, the code inside the if block is executed. Otherwise, the code inside the else block is executed. Here's an example:
const int temperatureThreshold = 30;
int temperature = 28;
void setup() {
// Initialization
}
void loop() {
if (temperature > temperatureThreshold) {
// High temperature, turn on fan
// Code for fan control
} else {
// Normal temperature, turn off fan
// Code for turning off fan
}
// Other code in the loop
}
In this example, we have a temperature variable and a temperatureThreshold constant representing a temperature threshold. Inside the loop() function, we use an if-else statement to check if the current temperature is greater than the threshold.
If the temperature is higher, the code inside the if block is executed, indicating that the fan should be turned on. If the temperature is not higher, the code inside the else block is executed, indicating that the fan should be turned off. The code for fan control or turning off the fan would be placed within the corresponding block.
For Loop
The for loop is used to repeat a block of code for a specific number of iterations. It consists of an initialization, a condition, and an increment or decrement. Here's an example:
const int ledPin = 9;
void setup() {
pinMode(ledPin, OUTPUT);
}
void loop() {
for (int i = 0; i < 5; i++) {
digitalWrite(ledPin, HIGH);
delay(500);
digitalWrite(ledPin, LOW);
delay(500);
}
// Other code in the loop
}
In this example, we have an LED connected to pin 9. Inside the loop() function, we use a for loop to blink the LED five times with a delay of 500 milliseconds between each state change.
The for loop has three parts:
Initialization (int i = 0) sets the initial value of the loop counter to zero.
Condition (i < 5) checks if the loop counter is less than 5.
Increment (i++) increments the loop counter by one after each iteration.
Inside the loop, the LED is turned on using digitalWrite() with HIGH and then turned off with LOW after a delay of 500 milliseconds using delay(). This process repeats five times, as specified by the loop condition.
While Loop
The while loop is used to repeat a block of code as long as a condition is true. Here's an example:
const int buttonPin = 2;
int buttonState = 0;
void setup() {
pinMode(buttonPin, INPUT);
}
void loop() {
buttonState = digitalRead(buttonPin);
while (buttonState == HIGH) {
// Code to execute when the button is pressed
// ...
buttonState = digitalRead(buttonPin);
}
// Other code in the loop
}
In this example, we have a push-button connected to pin 2. Inside the loop() function, we use a while loop to continuously check the state of the button.
First, we read the state of the button using digitalRead() and store it in the buttonState variable. The while loop then checks if the buttonState is HIGH, indicating that the button is pressed.
If the button is pressed, the code inside the while loop block is executed. You can include any desired actions to be performed when the button is pressed. After executing the code inside the while loop, the buttonState is again updated by reading the button state.
This process continues until the button is released (when the buttonState becomes LOW), and the program moves on to execute other code in the loop.
These examples illustrate the basic usage of if-else statements, for loops, and while loops in Arduino programming. Control structures allow you to make decisions and repeat actions, enabling more complex behaviors and interactivity in your Arduino projects.
Libraries
Arduino libraries are collections of pre-written code that extend the functionality of the Arduino platform. They provide ready-to-use functions for specific tasks, ranging from controlling LCD displays to connecting to Wi-Fi networks. Learning how to leverage libraries can save you time and effort in implementing complex functionalities.
Servo Library
The Servo library allows you to control servo motors easily. Servo motors are commonly used in robotics and various projects that require precise control of angular position. Here's an example:
#include
Servo myservo;
void setup() {
myservo.attach(9);
}
void loop() {
myservo.write(90); // Set servo to 90 degrees
delay(1000);
myservo.write(0); // Set servo to 0 degrees
delay(1000);
}
In this example, we include the Servo library using #include
In the loop() function, we use myservo.write() to set the servo's position. The value passed to write() represents the desired angle in degrees. In this case, we set the servo to 90 degrees and then to 0 degrees, with a delay of 1 second between each movement.
LiquidCrystal Library
The LiquidCrystal library enables communication with character LCD displays. Character LCDs are widely used for displaying text and simple graphics. Here's an example:
#include
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
void setup() {
lcd.begin(16, 2); // Initialize the LCD with 16 columns and 2 rows
lcd.print("Hello, World!");
}
void loop() {
// Other code in the loop
}
In this example, we include the LiquidCrystal library using #include
Inside the setup() function, we call lcd.begin() to initialize the LCD with 16 columns and 2 rows. Then, we use lcd.print() to display the text "Hello, World!" on the LCD.
WiFi Library (ESP8266WiFi or WiFiNINA):
The WiFi library allows Arduino boards to connect to wireless networks and perform various network-related operations. It is commonly used for IoT projects and applications that require internet connectivity. Here's an example using the ESP8266WiFi library:
#include
const char* ssid = "YourNetworkSSID";
const char* password = "YourNetworkPassword";
void setup() {
Serial.begin(9600);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi!");
}
void loop() {
// Other code in the loop
}
In this example, we include the ESP8266WiFi library using #include
Inside the setup() function, we initialize the serial communication using Serial.begin(). Then, we call WiFi.begin() and pass the SSID and password to connect to the WiFi network.
The while loop continuously checks the connection status using WiFi.status(). It delays for 1 second and prints a message until the connection is established (WL_CONNECTED).
Once the connection is established, the program proceeds, and you can add other code to perform network-related tasks or interact with web services.
DHT Library
The DHT library allows you to interface with DHT series temperature and humidity sensors, such as DHT11 or DHT22. It provides functions to read sensor data accurately. Here's an example using the DHT library:
#include
#define DHTPIN 2
#define DHTTYPE DHT11
DHT dht(DHTPIN, DHTTYPE);
void setup() {
Serial.begin(9600);
dht.begin();
}
void loop() {
float temperature = dht.readTemperature();
float humidity = dht.readHumidity();
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.print("°C, Humidity: ");
Serial.print(humidity);
Serial.println("%");
delay(2000);
}
In this example, we include the DHT library using #include
Inside the setup() function, we initialize the serial communication using Serial.begin(), and then call dht.begin() to initialize the DHT sensor.
In the loop() function, we use dht.readTemperature() to read the temperature in Celsius and dht.readHumidity() to read the humidity percentage.
We print the temperature and humidity values to the serial monitor using Serial.print() and Serial.println(). The program then delays for 2 seconds (2000 milliseconds) before repeating the process.
Adafruit NeoPixel Library
The Adafruit NeoPixel library enables control of individually addressable RGB LED strips and rings, such as the popular WS2812 or SK6812 LEDs. Here's an example:
#include
#define LED_PIN 6
#define LED_COUNT 8
Adafruit_NeoPixel strip(LED_COUNT, LED_PIN, NEO_GRB + NEO_KHZ800);
void setup() {
strip.begin();
strip.show(); // Initialize all pixels to off
}
void loop() {
// Set pixel colors
strip.setPixelColor(0, 255, 0, 0); // Red
strip.setPixelColor(1, 0, 255, 0); // Green
strip.setPixelColor(2, 0, 0, 255); // Blue
strip.show(); // Update the LED strip
delay(1000);
}
In this example, we include the Adafruit NeoPixel library using #include
We create an instance of the Adafruit_NeoPixel class called strip, specifying the LED count and pin.
Inside the setup() function, we call strip.begin() to initialize the NeoPixel strip. Then, we use strip.show() to turn off all the LEDs at the start.
In the loop() function, we use strip.setPixelColor() to set the color of individual pixels. We pass the pixel index and the RGB values (red, green, blue) to specify the desired color. In this case, we set the first pixel to red, the second to green, and the third to blue.
Finally, we call strip.show() to update the LED strip with the new colors, and then add a delay of 1 second.These examples demonstrate how libraries can simplify the integration of complex functionalities and hardware components into your Arduino projects. By utilizing libraries, you can leverage existing code and functions, enabling you to focus on higher-level application logic and functionalities.
Serial Communication
Serial communication enables the Arduino board to communicate with other devices, such as computers, via the Universal Serial Bus (USB). By utilizing the Serial object and functions like Serial.begin() and Serial.println(), you can send and receive data for debugging, data logging, or interaction with external software.
Arduino serial programming allows communication between an Arduino board and a computer or other devices using a serial interface. It enables sending and receiving data in a serial manner, which is useful for debugging, data logging, controlling the Arduino remotely, and interfacing with other devices. Let's explore Arduino serial programming with some examples:
Sending Data to Serial Monitor
void setup() {
Serial.begin(9600);
}
void loop() {
int sensorValue = analogRead(A0);
Serial.print("Sensor Value: ");
Serial.println(sensorValue);
delay(1000);
}
In this example, we initialize the serial communication using Serial.begin(9600) in the setup() function. The baud rate is set to 9600 bits per second.
Inside the loop() function, we read the analog value from pin A0 using analogRead() and store it in the sensorValue variable.
We then use Serial.print() to send the text "Sensor Value: " to the serial monitor, followed by Serial.println() to send the actual sensor value. The println() function adds a newline character after printing the value.
The program delays for 1 second using delay(1000) before repeating the process. Open the serial monitor in the Arduino IDE to view the output.
Receiving Data from Serial Monitor
void setup() {
Serial.begin(9600);
}
void loop() {
if (Serial.available() > 0) {
char receivedChar = Serial.read();
Serial.print("Received Character: ");
Serial.println(receivedChar);
}
}
In this example, we initialize the serial communication using Serial.begin(9600) in the setup() function.
Inside the loop() function, we use the Serial.available() function to check if there are any incoming data bytes available in the serial buffer.
If data is available, we read a single character using Serial.read() and store it in the receivedChar variable.
We then use Serial.print() to send the text "Received Character: " to the serial monitor, followed by Serial.println() to send the received character value. The println() function adds a newline character after printing the value.
The program continuously loops and checks for incoming data from the serial monitor. When a character is entered in the serial monitor, it will be received and displayed.
The above examples demonstrate basic usage of Arduino serial programming. It allows bidirectional communication between the Arduino board and a connected device or computer through the serial interface. You can send data from the Arduino to the serial monitor or receive data from the serial monitor and perform actions based on the received data. Serial programming is a powerful tool for interacting with and monitoring your Arduino projects.
Arduino programming offers an exciting gateway into the world of electronics and physical computing. By grasping the basic concepts discussed in this primer, you are well on your way to unleashing your creativity and building innovative projects. Remember to experiment, practice, and explore the vast resources available in the Arduino community to deepen your understanding and expand your programming skills. Happy coding!
Unlock the Secrets: Get Your Copy Now!
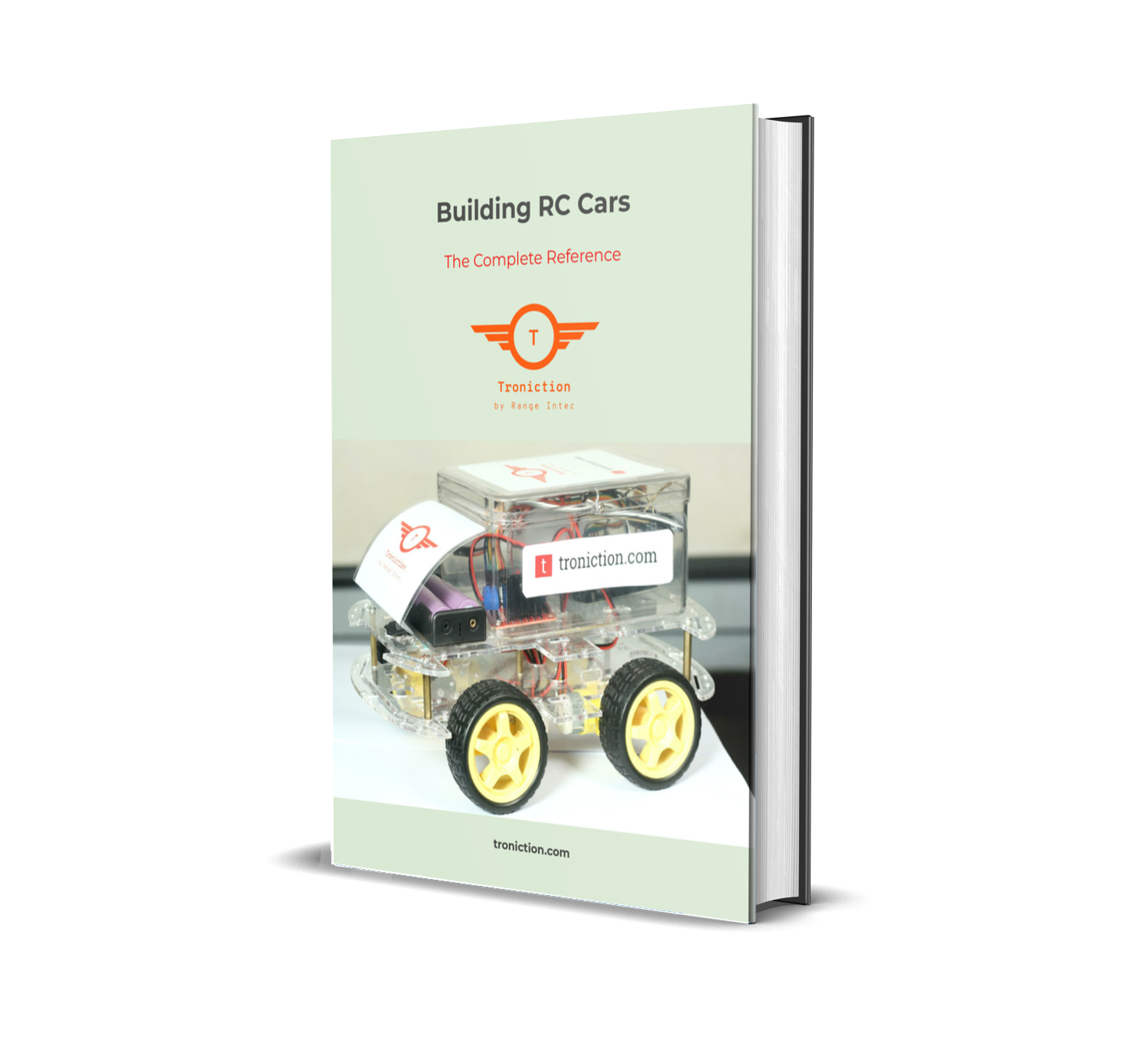
Discover the ultimate resource for building your very own remote-controlled Arduino-based electronic car. Download the comprehensive PDF file that contains everything you need to know, right at your fingertips.
Get the Book Now!